About matplotlib
Matplotlib is a python plotting library which can help generate plots, and charts.
Installation
Install python3 matplotlib on my Ubuntu 16.04 box
1 | $ sudo apt install python3-matplotlib |
Creating a simple chart in python shell
1 | $ python3 |
Then I got the chart
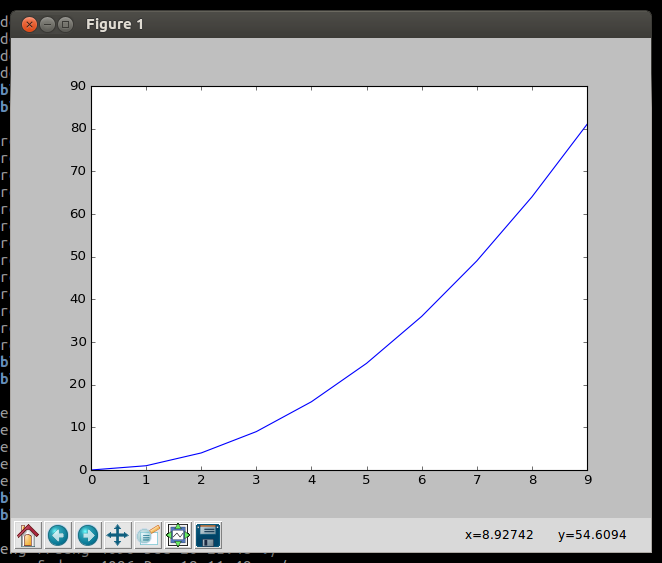
Creating a bar chart
Next I wrote a script to generate a bar chart of the average monthly high temperature of San Francisco.
1 | import matplotlib.pyplot as plt |
Then I got the bar chart
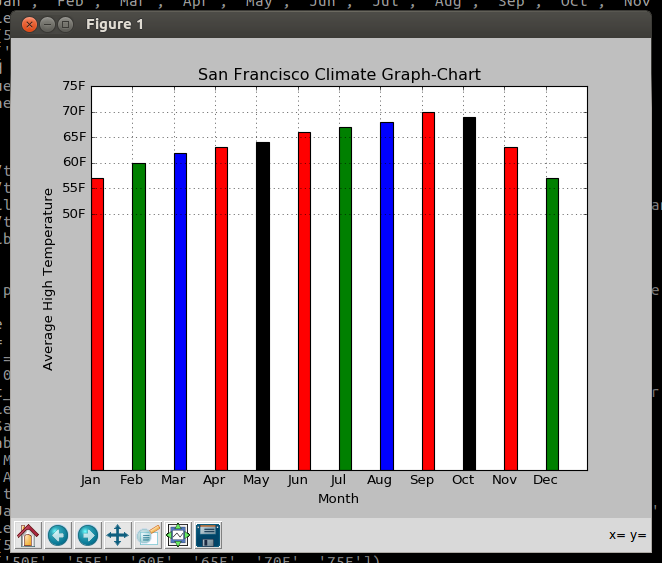
Creating a pie chart
This script I wrote generates a pie chart of the Population for California by race in 2017 and 2016
1 | import matplotlib.pyplot as plt |
The pie chart I got
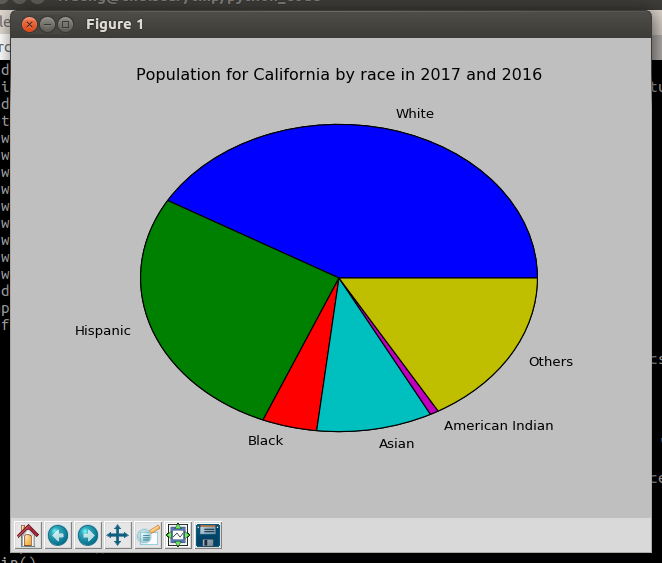
Save the chart to a image file
Just use the savefig() method.
1 | plt.savefig('bar_chart.png') |